A complete guide for technical developers: From RTSP protocol principles to SmolRTSP implementation in embedded systems
1. Introduction: Importance of RTSP Protocol in Embedded Systems
With the rapid growth of the Internet of Things (IoT) and smart devices, real-time audio and video transmission has become increasingly vital in embedded systems. Whether for smart cameras, drones, or industrial monitoring equipment, efficient, low-latency streaming solutions are essential. Among various protocols, RTSP (Real-Time Streaming Protocol) is preferred for its flexibility and broad support in implementing streaming in embedded systems.
2. Overview of RTSP Protocol
2.1 What is RTSP?
RTSP is an application-layer protocol designed to control streaming media servers. It allows clients to send commands like "play," "pause," and "stop" to control audio and video streams in real-time. Note that RTSP itself does not transport media data; it uses RTP (Real-time Transport Protocol) for data transmission and RTCP (Real-time Control Protocol) for control information.
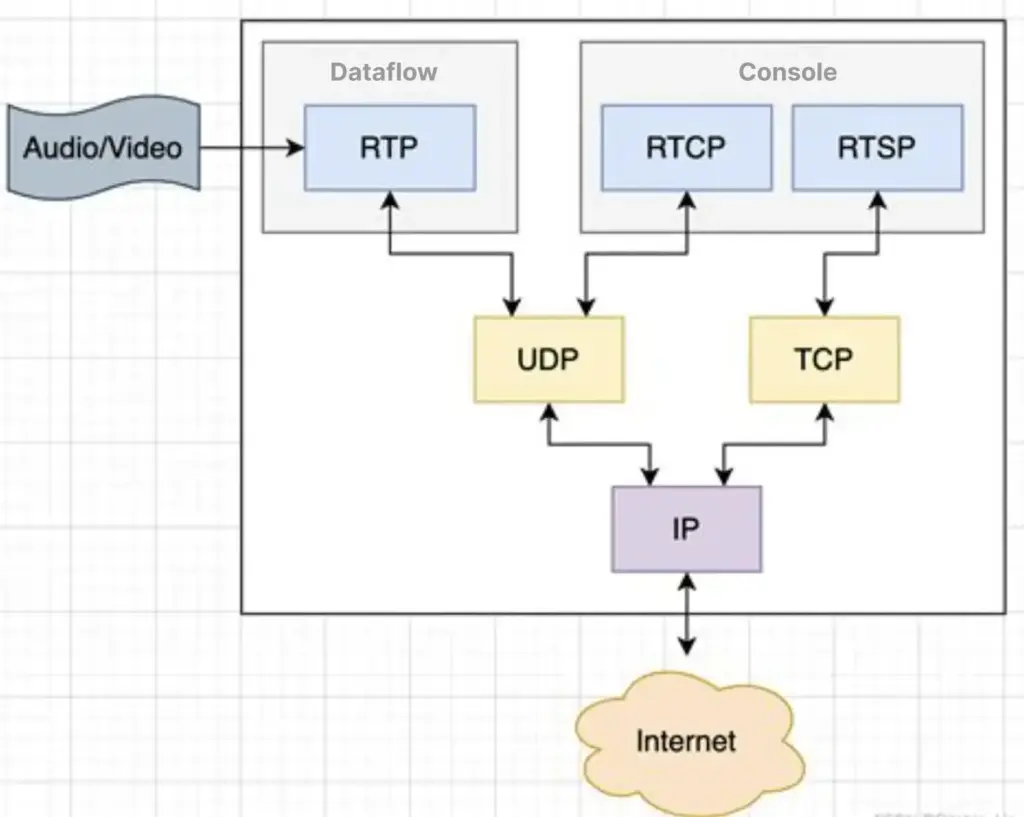
2.2 How Does RTSP Work
RTSP uses a client-server model, and its communication typically involves the following steps:
- OPTIONS: The client queries the server for supported commands.
- DESCRIBE: The client requests media description information, usually returned in SDP (Session Description Protocol) format.
- SETUP: The client requests to establish a transport channel for the media stream.
- PLAY: The client requests to start streaming the media.
- PAUSE: The client requests to pause the media stream.
- TEARDOWN: The client requests to terminate the media stream.
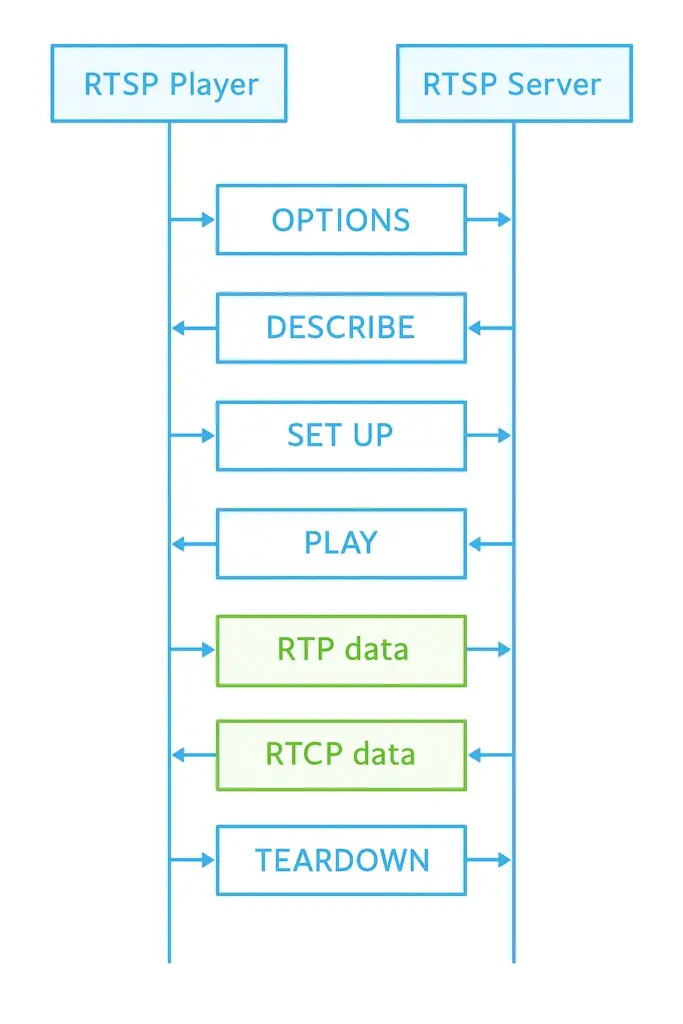
These commands allow clients to flexibly control media playback, enabling functions like fast forward, pause, and stop.
2.3 How To Use RTSP Protocol in Browsers
Using the RTSP (Real-Time Streaming Protocol) in browsers can be challenging since most modern web browsers do not natively support RTSP streams. However, there are several methods you can use to enable RTSP streaming in a browser:
- Use a Media Server: Convert RTSP streams to a format supported by browsers, such as HLS (HTTP Live Streaming) or WebRTC. Media servers like Wowza, Red5, or Ant Media Server can perform this conversion.
- HTML5 Video Player with Plugins: Utilize HTML5 video players with specific plugins or extensions that support RTSP streams. Some players offer plugins that can handle RTSP or integrate with third-party services.
- Browser Extensions: Some browser extensions or add-ons can enable RTSP streaming by acting as a bridge between the RTSP source and the browser.
- Custom Web Applications: Develop custom web applications using libraries that support RTSP streaming. Libraries such as JSMpeg or video.js can be used in conjunction with a backend service to handle RTSP streams.
- Use VLC Plugin: Although less common due to security and compatibility issues, using the VLC web plugin can allow RTSP playback in browsers that support it.
By implementing these methods, you can effectively stream RTSP content in a browser environment, providing users with seamless access to real-time video streams.
2.4 Challenges of RTSP in Embedded Systems
Implementing an RTSP server in embedded systems faces several challenges:
- Resource Constraints: Embedded devices typically have limited processing power and memory, making it difficult to run resource-intensive RTSP servers.
- High Real-Time Requirements: Audio and video streaming demands strict latency and synchronization.
- Protocol Complexity: RTSP involves multiple commands and state management, making implementation complex.
Thus, a lightweight and easy-to-implement RTSP server solution is needed to meet the demands of embedded systems.
3. SmolRTSP: A Lightweight RTSP Server for Embedded Systems
SmolRTSP is a lightweight server library compliant with the RTSP 1.0 standard, designed specifically for embedded devices. It supports TCP and UDP transport, allows any data payload format, and provides a flexible API for developers to implement RTSP functionality in resource-constrained environments.
3.1 Features of SmolRTSP
- Lightweight: The core library includes only necessary features, suitable for the resource limitations of embedded devices.
- Easy Integration: Offers clear API interfaces for seamless integration with existing systems.
- High Performance: Optimized data processing ensures low-latency media streaming.
- Open Source: Licensed under MIT, encouraging community contributions and custom development.
3.2 Applications of SmolRTSP
SmolRTSP is suitable for various embedded system scenarios, including but not limited to:
- Smart Cameras: Enable remote access and control of real-time video streams.
- Drones: Transmit real-time aerial video streams.
- Industrial Monitoring Equipment: Facilitate remote monitoring and control functions.
- Home Automation Systems: Integrate video surveillance features.
4. SmolRTSP Architecture and Module Analysis
SmolRTSP is designed as a modular, low-resource, highly customizable RTSP service library. Its core follows principles of simplicity and practicality, suitable for running on bare-metal or embedded Linux systems.
Below is a typical architecture of SmolRTSP:
graph TD Client[RTSP Client] -->|TCP/UDP| SmolRTSP[SmolRTSP Server] SmolRTSP --> Parser[RTSP Parsing Module] SmolRTSP --> Dispatcher[Command Dispatch Module] SmolRTSP --> SessionManager[Session Manager] SmolRTSP --> RTPStack[RTP Sending Module] RTPStack --> EncodedStream[Encoded Video/Audio Stream]
4.1 Detailed Core Modules
✅ RTSP Parsing Module (Parser)
• Receives RTSP requests from clients (e.g., DESCRIBE, SETUP, PLAY)
• Parses RTSP messages using a state machine
• Supports standard RTSP 1.0 protocol format and extended SDP (Session Description Protocol)
✅ Command Dispatch Module (Dispatcher)
• Calls the corresponding handler functions based on different RTSP commands
• Supports custom handlers, such as hooks to the application layer for dynamic control of streaming/recording
✅ Session Manager (SessionManager)
• Tracks client states, including session_id, channel, port, etc., after SETUP
• Supports concurrent connections from multiple clients (relies on underlying task scheduler or select/poll)
✅ RTP Sending Module (RTPStack)
• Constructs RTP packets and pushes them to clients via UDP/TCP at fixed intervals
• Adapts to mainstream video encoding formats like H264/H265 (requires external encoder support)
5. Deploying SmolRTSP on Embedded Platforms
5.1 Compilation Dependencies and Resource Requirements
SmolRTSP is written in Rust, requiring the following toolchain support:
• Rust compiler (can use cross for cross-compilation)
• libc / musl toolchain (depending on the platform)
• Minimum memory usage: ≈ 100KB (depending on feature trimming)
📌 For mainstream embedded SoCs like STM32MP1, Allwinner V851, and RK3588S, SmolRTSP can run smoothly within 256MB of memory.
5.2 Typical Integration Methods
Integration Scenario | Description | Interface Method |
---|---|---|
🧠 Integration with Proprietary Video Encoder | Pass frame buffers, SmolRTSP handles RTP packaging and pushing | Provide raw frame interface (YUV/H264 buffer) |
🎥 Integration with Camera Driver | Video capture thread pushes frames in real-time | Use mmap/V4L2 to capture frames and send to SmolRTSP |
🎯 Collaboration with Media Server (e.g., FFmpeg) | Acts as upstream streaming server for FFmpeg/OBS to pull streams | Directly listen to socket, standard SDP description support |
📡 Simultaneous WebRTC/RTMP Streaming | Parallel streaming with other protocols | Reuse the same video capture layer, register socket for pushing |
5.3 Sample Integration Code (Embedded Pseudo Code)
fn start_streaming() {
// Initialize the camera
let video_capture = V4l2Capture::new("/dev/video0");
// Start the SmolRTSP server
let server = SmolRTSPServer::bind("0.0.0.0:8554");
loop {
// Read a frame
let frame = video_capture.read_frame();
// Encode as H264 (assuming software encoding)
let encoded = h264_encode(frame);
// Push to RTSP session
server.broadcast_rtp(encoded);
}
}
📌 Note: SmolRTSP itself does not include an H264 encoder; external libraries (e.g., x264, OpenH264, FFmpeg) are required for encoding.
5.4 Embedded Debugging Tips
Issue | Cause | Debugging Method |
---|---|---|
No data after client connection | broadcast_rtp not called correctly / session not established | Print SessionManager status, confirm if SETUP is complete |
Playback black screen or stuttering | Timestamp errors / I-frame loss / encoder issues | Use Wireshark to capture packets + FFplay to compare latency |
Compilation failure | Rust toolchain mismatch | Use rustup target add to install cross-compilation target |
6. Comparison with Other RTSP Servers
When choosing an RTSP service framework for embedded systems, developers face several options, such as Live555, EasyRTSPServer, and FFserver. How does SmolRTSP compare in terms of advantages or limitations?
Project | SmolRTSP | Live555 | EasyRTSPServer | FFserver |
---|---|---|---|---|
Language | Rust | C++ | C++ | C |
Memory Usage | ≈ 100–200KB | 1MB+ | 5MB+ | Discontinued |
Embedded Suitability | ✅ Excellent | Moderate (requires trimming) | Heavy | ❌ Not recommended |
Development Flexibility | ✅ Fully customizable streams | ❌ Heavy on general API | ⚠️ Fixed stream structure | ❌ Maintenance stopped |
RTP Sending Performance | Moderate to high | Excellent | Excellent | Moderate |
Encoder Dependency | None (requires external) | Built-in support for some | Built-in | Built-in |
Multi-Protocol Support | RTSP only | Supports full RTCP/RTP link | Supports RTMP extension | Supports various (but not maintained) |
📌 Conclusion: If you are working on embedded systems, are sensitive to resource usage, and want high customization, SmolRTSP is a great choice. However, if you need ready support for RTMP / HLS / HTTPS and other protocols, Live555 may be more suitable.
7. Performance Optimization Suggestions and Production Practices
7.1 SmolRTSP Performance Bottleneck Analysis
Bottleneck | Cause | Optimization Suggestions |
---|---|---|
RTP Latency Fluctuations | Unstable timer / network jitter | Use timer thread + high-priority socket |
High Encoding Overhead | Inefficient software encoder | Use hardware H264 encoder (e.g., VENC) |
Session Context Memory Usage | Accumulation with many clients | Limit maximum connections + timeout recovery |
Frequent Context Switching | IO/encoding not decoupled | Use asynchronous + single-threaded data pipeline structure |
7.2 Recommended Practice Scenarios
Application Scenario | Description | Recommended Configuration |
---|---|---|
🏠 Home Smart Cameras | Plug-in cameras/battery doorbells | Use V4L2 + YUV capture + SmolRTSP |
🚁 Drone Video Transmission System | Real-time stream transmission | Integrate hardware encoding + custom SDP |
🏭 Industrial Inspection Terminals | Multi-channel image upload | Multi-process collaborative streaming, each with an independent socket |
🐕 Pet Feeder/Visual Door Lock | Embedded edge video | Single-threaded minimalist push architecture (frame rate ≤15) |
7.3 Future Expansion Directions for SmolRTSP
• ✅ Support ONVIF / RTSP over TLS
• ✅ Simplify SDP generation, compatible with more clients (e.g., VLC, FFplay, Hikvision SDK)
• ✅ Implement Web embedded streaming with Rust + WASM
• ✅ Provide turn-key framework with hardware platforms (e.g., Raspberry Pi, ESP32-S3)
8. Developer Recommendations
"If you want to run an efficient, customizable RTSP server on embedded systems, rather than using traditional heavy server frameworks—SmolRTSP is worth trying."
Pros | Cons |
---|---|
✅ Minimal design, easy to embed | ❌ No encoder, requires external H264 support |
✅ Fully open-source, flexible interface | ❌ Lacks UI management interface (requires command-line debugging) |
✅ Low resource usage, suitable for edge devices | ❌ Documentation is relatively brief, requires source code for understanding architecture |
🧰 Engineering Recommendations:
• Use cross-compilation for Rust projects when integrating, recommended to use cross
• For encoding, consider using FFmpeg CLI or OpenH264 SDK
• For multi-streaming, implement asynchronous concurrency with tokio or async-std
📎 Project Links:
GitHub: [GitHub - OpenIPC/smolrtsp: A lightweight real-time streaming library for IP cameras