With the increasing prevalence and complexity of IoT devices, the demand for advanced embedded development tools has grown significantly. Traditional embedded development often relies on C or similar low-level languages, which not only have a steep learning curve but also limit development efficiency and code maintainability. To address these challenges, Microsoft introduced DeviceScript, a TypeScript-based tool tailored for resource-constrained microcontroller devices.
DeviceScript brings the modern development experience of TypeScript to embedded systems, simplifying the development process, supporting multiple hardware protocols, and enhancing code readability and reusability. This offers IoT developers a fresh and efficient alternative.
What is DeviceScript?
DeviceScript is an open-source project developed by Microsoft Research, specifically designed for low-power, low-storage, and resource-constrained embedded devices. Its core idea is to run a small virtual machine on these devices, compiling TypeScript into bytecode that executes directly on microcontrollers. This approach enables developers to enjoy a modern programming language in an embedded environment.
Key Features
- TypeScript Support
Program using the popular TypeScript language with familiar syntax and tooling. - Lightweight Virtual Machine
A compact virtual machine designed for efficient operation on low-power microcontrollers. - Hardware Abstraction Services
Abstracted hardware interfaces allow developers to quickly build cross-device IoT applications. - Modular Ecosystem Support
Integration with npm, yarn, and other package management tools enables developers to reuse existing modules for rapid application development.
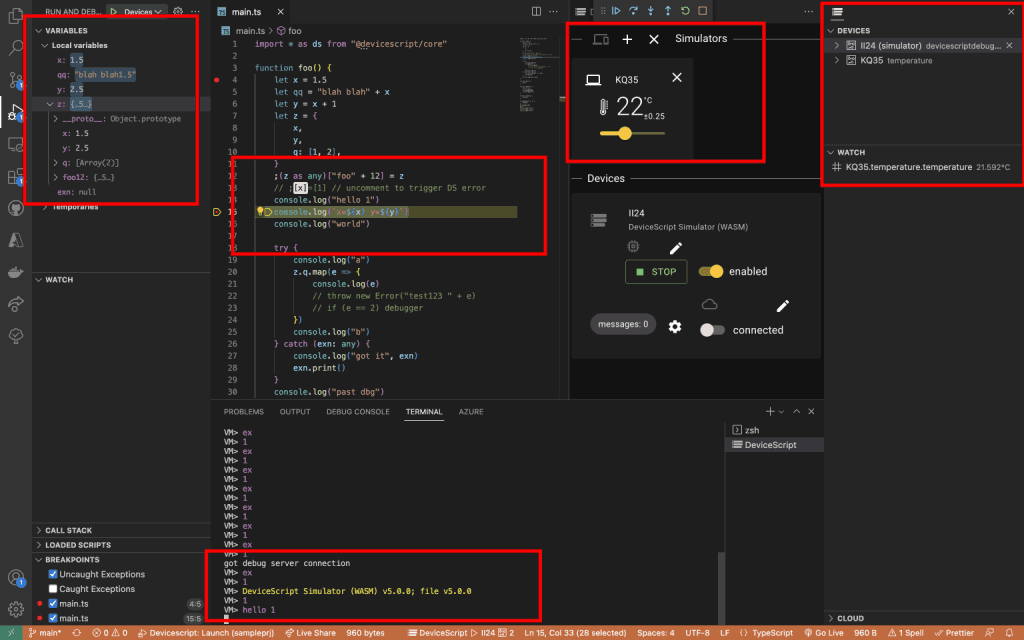
Technical Architecture
DeviceScript's architecture can be divided into the following layers:
- Language Layer: Developers write logic using TypeScript, benefiting from static type checking and modern language features.
- Compilation Layer: The DeviceScript compiler converts TypeScript code into bytecode.
- Runtime Layer: The microcontroller runs the DeviceScript virtual machine (VM), interpreting and executing bytecode.
- Hardware Layer: The virtual machine interacts with the hardware through abstraction interfaces to control devices and collect data.
Architecture Diagram
graph TB subgraph "Development Environment" A[TypeScript Source Code] A --> B[DeviceScript Compiler] B --> C[Bytecode Generation] end subgraph "Deployment Environment" C --> D[DeviceScript Virtual Machine] D -->|Hardware Abstraction Interface| E[Underlying Hardware] E --> F[External Devices (Sensors, Actuators)] end subgraph "Supported Hardware Platforms" E --> E_connector[Hardware Support] E_connector --> I[ESP32 Series] E_connector --> J[RP2040 Series] E_connector --> K[Other Supported Devices] end subgraph "Debugging and Feedback" A --> G[Visual Studio Code Debugging] G -->|Real-time Feedback| B D -->|Data Stream| H[Logs and Status Feedback] H --> G end
Explanation:
- Developers use TypeScript for high-level development, avoiding the complexity of low-level hardware details.
- The compiler generates optimized bytecode for efficient execution.
- The virtual machine interprets the bytecode and interacts with devices through abstraction interfaces.
Main Features of DeviceScript
1. TypeScript-Driven Development Experience
Compared to traditional C/C++ for embedded development, DeviceScript offers a modern programming language with enhanced features:
- Static Type Checking: Reduces common runtime errors.
- Async/Await Programming: Simplifies implementation of complex event-driven logic.
- Modular Design: Supports npm packages for improved development efficiency.
For example, the following code demonstrates how to control an LED to blink:
import { delay } from "@devicescript/core";
import { setStatusLight } from "@devicescript/runtime";
setInterval(async () => {
await setStatusLight(0); // Turn off LED
await delay(1000);
await setStatusLight(0x0f0f0f); // Turn on LED
await delay(1000);
}, 10);
This code highlights TypeScript's simplicity and powerful asynchronous capabilities.
2. Support for Multiple Hardware Platforms
DeviceScript supports the following mainstream microcontroller platforms:
- ESP32 Series: Including ESP32, ESP32-S2, ESP32-S3, ESP32-C3, and more.
- RP2040 Series: Such as Raspberry Pi Pico.
These platforms are widely used in IoT projects. With DeviceScript, developers can implement more sophisticated application logic on this hardware.
Current Support Matrix
Device | USB | TCP | TLS | I2C | SPI | GPIO | PWM | WS2812B | Jacdac |
---|---|---|---|---|---|---|---|---|---|
WASM Sim | N/A | ✓ | ✓ | ❌ | ❌ | ❌ | ❌ | ❌ | ✓ |
ESP32 | ⚠️ | ✓ | ✓ | ✓ | ✓ | ✓ | ✓ | ✓ | ✓ |
ESP32-C3 | ✓ | ✓ | ✓ | ✓ | ✓ | ✓ | ✓ | ✓ | ✓ |
ESP32-S2 | ✓ | ✓ | ❌ | ✓ | ✓ | ✓ | ✓ | ✓ | ✓ |
ESP32-S3 | ✓ | ✓ | ✓ | ✓ | ✓ | ✓ | ✓ | ✓ | ✓ |
RP2040 | ✓ | ❌ | ❌ | ✓ | ✓ | ✓ | ✓ | ❌ | ✓ |
RP2040-W | ✓ | ✓ | ❌ | ✓ | ✓ | ✓ | ✓ | ❌ | ✓ |
3. Hardware Abstraction and Cross-Platform Development
DeviceScript provides a unified hardware abstraction interface. Whether the target device is ESP32 or RP2040, developers can control hardware using the same API. This cross-platform capability greatly enhances code portability and maintainability.
For example, the following code controls a temperature sensor on any supported hardware:
import { TemperatureSensor } from "@devicescript/devices";
const tempSensor = new TemperatureSensor();
setInterval(async () => {
console.log(`Temperature: ${await tempSensor.readTemperature()}°C`);
}, 1000);
Regardless of whether the sensor uses I2C, SPI, or UART protocols, DeviceScript handles the underlying details, allowing developers to focus on higher-level logic.
4. Simulation and Debugging Support
DeviceScript integrates robust debugging tools within Visual Studio Code, supporting testing in both hardware and simulated environments. This efficient debugging capability enables developers to quickly identify and resolve issues without running code on actual hardware each time.
Development Workflow with DeviceScript
DeviceScript's development and deployment workflow is straightforward and efficient, involving the following steps:
- Write Code
Use TypeScript to write device logic, leveraging modularity and hardware abstraction interfaces to quickly implement features. - Compile to Bytecode
Compile TypeScript source code into bytecode using DeviceScript's compilation toolchain. - Deploy to Device
Flash the bytecode and DeviceScript virtual machine firmware onto supported hardware devices. - Execute and Debug
Once the device starts, the DeviceScript virtual machine interprets and executes the bytecode. Developers can remotely debug via Visual Studio Code.
Workflow Diagram
flowchart LR A[TypeScript Code] --> B[DeviceScript Compiler] B --> C[Bytecode] C --> D[Deploy to Device] D --> E[DeviceScript Virtual Machine] E --> F[Hardware Execution] F --> G[Real-World Actions]
Practical Applications of DeviceScript
1. Rapid Prototyping
DeviceScript offers an efficient development experience, making it ideal for rapid prototyping. Developers can leverage existing npm modules and hardware abstraction interfaces to quickly implement IoT device functionality and deploy it directly to the target device after debugging.
Example: Develop an indoor environment monitoring device based on ESP32. By connecting temperature, humidity, and gas sensors, the device can collect data in real-time and upload it to the cloud.
2. IoT Sensor Nodes
DeviceScript simplifies the logic design for low-power devices such as sensor data collection and wireless transmission. Using asynchronous programming, developers can optimize the device’s power consumption and response time.
Example: In an agricultural application, use an RP2040 microcontroller to monitor soil moisture and transmit the data to a gateway via the LoRa communication protocol.
3. Smart Home Devices
DeviceScript simplifies the development process for smart home devices such as smart switches and lighting controllers. Developers can use the hardware abstraction layer to quickly implement complex automation logic.
Example: Create a voice-controlled smart light bulb that interacts with a voice assistant over WiFi and supports different lighting modes for various scenarios.
4. Education and Learning
For beginners and educational institutions, DeviceScript serves as an excellent teaching tool. It lowers the barrier to entry for embedded development, enabling students to quickly grasp the design and implementation of IoT devices.
Example: Teach programming using Raspberry Pi Pico, showing students how to use TypeScript to build a simple IoT temperature monitoring system.
Advantages of DeviceScript
1. Development Efficiency
DeviceScript uses TypeScript, providing a high-efficiency development environment. Developers can utilize modern language features like asynchronous functions and static type checking to build and debug projects quickly.
2. Cross-Platform Capability
With hardware abstraction services, DeviceScript ensures high code reusability, allowing the same codebase to run on devices across different platforms.
3. Modularity and Ecosystem
DeviceScript supports integration with npm modules, offering developers a wide range of extensions for functions such as data analysis and communication protocol encapsulation.
4. Beginner-Friendly
For developers with little experience in embedded development, DeviceScript’s ease of use makes it an ideal starting tool. At the same time, it supports complex application development to meet the needs of advanced users.
Conclusion
DeviceScript is a highly promising modern embedded development tool. By introducing TypeScript, it offers a high-efficiency, intuitive development experience for resource-constrained microcontroller devices. Its support for cross-platform and modular design significantly lowers the barrier to entry for IoT development.
Whether for rapid prototyping, IoT sensor node development, smart home applications, or educational use, DeviceScript provides flexible solutions. As an open-source tool, it offers IoT developers a fresh choice and showcases the future direction of embedded development.
If you are interested in DeviceScript, you can explore its official documentation or GitHub repository to start discovering its powerful features!