Serial Studio is a versatile, cross-platform software designed for embedded engineers, IoT developers, hobbyists, and educators. It simplifies the process of visualizing real-time data from serial ports, MQTT, Bluetooth, and network sockets, eliminating the need to create custom visualization tools for each application. This blog introduces Serial Studio's key features, its technical advantages, and real-world use cases, accompanied by diagrams, tables, and source code examples.
1. Why Serial Studio?
In the world of embedded systems and IoT, data visualization is crucial for understanding system behavior, debugging, and presenting results. Serial Studio streamlines this process by providing:
- Cross-platform compatibility: Works seamlessly on Windows, macOS, and Linux.
- Support for multiple data sources: Serial ports, MQTT, BLE, and TCP/UDP.
- Customizable dashboards: Tailored visualization for specific applications.
2. Key Features of Serial Studio
2.1 Cross-Platform Compatibility
Serial Studio is available for Windows, macOS, and Linux, ensuring accessibility for developers regardless of their preferred operating system. This makes it ideal for collaborative projects where team members use different platforms.
2.2 Multiple Data Sources
Serial Studio supports a variety of data input methods:
- Serial Ports: USB or RS232 for direct hardware communication.
- MQTT: Internet-based real-time data exchange.
- Bluetooth Low Energy (BLE): For wireless IoT devices.
- TCP/UDP: Network socket communication for distributed systems.
2.3 Customizable Dashboards
Using the project editor, users can create tailored dashboards to represent data visually. Available widgets include:
- Graph plots: For real-time data trends.
- Gauge meters: To display thresholds and limits.
- Tables: For structured data visualization.
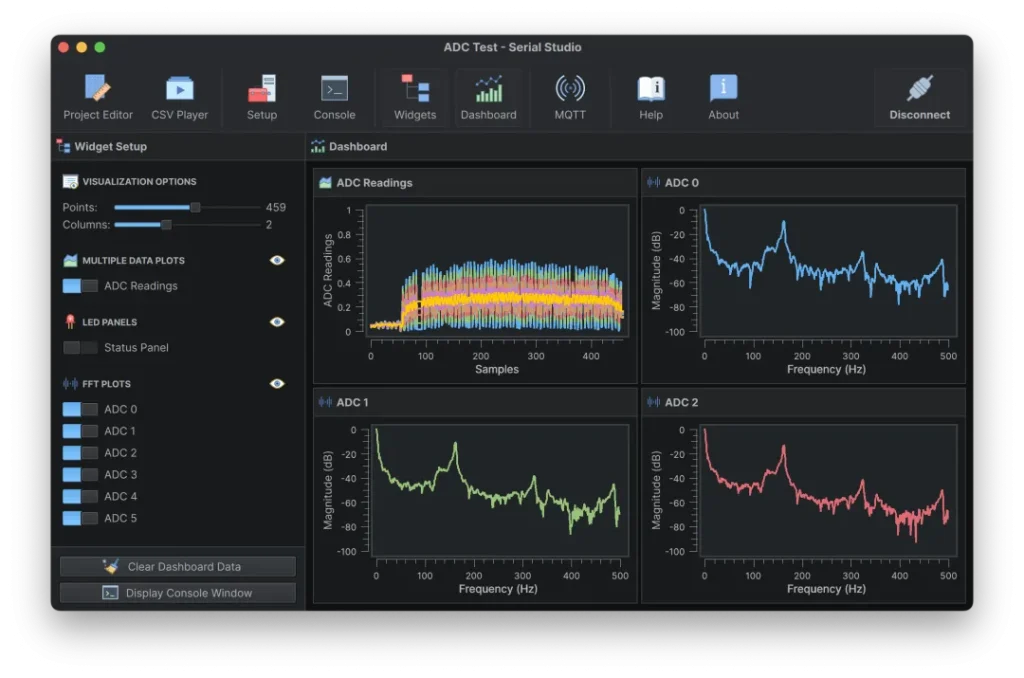
Example: Dashboard for an IoT Weather Station
widgets:
- type: "gauge"
name: "Temperature"
min: -20
max: 50
- type: "plot"
name: "Humidity Trend"
x_axis: "Time"
y_axis: "Humidity (%)"
- type: "table"
name: "Sensor Data"
columns: ["Timestamp", "Temperature", "Humidity"]
2.4 CSV Export
Serial Studio allows users to save received data in CSV format, enabling offline analysis and integration with tools like Excel or Python.
| Feature | Benefit | | | | | CSV Export | Enables long-term data storage | | Data Analysis | Compatible with analytics tools |
2.5 MQTT Support
Serial Studio's integration with MQTT makes it ideal for IoT applications. Developers can publish and receive data over the internet, enabling remote monitoring and control.
3. Technical Architecture
Serial Studio follows a modular architecture to ensure flexibility and scalability. Below is an overview of its core components:
3.1 Data Acquisition Layer
This layer is responsible for receiving data from hardware devices or network interfaces.
graph TD A[Device Sensors] --> B[Serial Port] A --> C[MQTT Broker] A --> D[BLE Module]
3.2 Processing Layer
Data is processed and formatted according to the user-defined structure.
3.3 Visualization Layer
The final layer displays the processed data on the dashboard using widgets.
4. Getting Started with Serial Studio
4.1 Installation
You can download the latest release of Serial Studio from its GitHub repository. It supports:
- Windows: An executable installer for quick setup.
- macOS: A ready-to-use application package.
- Linux: AppImage or package-based installation.
Installation Example (Linux)
# Download AppImage
wget https://github.com/Serial-Studio/Serial-Studio/releases/download/v1.0.0/Serial-Studio.AppImage
# Make it executable
chmod +x Serial-Studio.AppImage
# Run the application
./Serial-Studio.AppImage
4.2 Setting Up Your First Project
- Connect Your Device: Plug in your hardware (e.g., an Arduino with sensors) via USB or connect to a network interface.
- Configure Data Input: Select the appropriate data source (e.g., COM port for serial).
- Design the Dashboard: Use the project editor to define widgets.
- Start Visualization: Stream data in real-time and monitor outputs.
4.3 Example: Visualizing Temperature Data from Arduino
Here’s a basic example of using Serial Studio with an Arduino to monitor temperature data.
Arduino Code
void setup() {
Serial.begin(9600); // Initialize serial communication
}
void loop() {
float temperature = analogRead(A0) * 0.488; // Example sensor calculation
Serial.println(temperature);
delay(1000); // Send data every second
}
Serial Studio Dashboard Configuration
widgets:
- type: "gauge"
name: "Temperature"
min: 0
max: 100
unit: "°C"
Output Example
| Time (s) | Temperature (°C) | | | | | 0 | 25.5 | | 1 | 26.1 | | 2 | 25.8 |
5. Advanced Features and Use Cases
While Serial Studio simplifies basic data visualization, it also provides powerful advanced features for professional applications. This section explores these features and their practical implementations.
5.1 Advanced Features
5.1.1 Multi-Source Integration
Serial Studio supports simultaneous data streams from multiple devices or sources. This is particularly useful in systems requiring comprehensive monitoring across diverse components.
Example: Monitoring an IoT Home Automation System
- Source 1: MQTT broker for temperature and humidity data.
- Source 2: Serial port for motion sensor data.
- Source 3: BLE for wearable health trackers.
Multi-Source Data Flow
graph TD A[MQTT Broker] --> B[Serial Studio] C[Serial Port Device] --> B D[BLE Device] --> B B --> E[Unified Dashboard]
5.1.2 Widget Customization
Serial Studio allows for in-depth customization of widgets to suit specific visualization needs. Users can:
- Adjust color schemes for better readability.
- Modify widget dimensions for compact layouts.
- Implement conditional formatting to highlight anomalies.
Example: Conditional Formatting for Alerts
widgets:
- type: "gauge"
name: "CPU Temperature"
min: 0
max: 100
unit: "°C"
thresholds:
- value: 70
color: "red"
- value: 50
color: "yellow"
5.2 Real-World Use Cases
5.2.1 Industrial IoT Monitoring
Serial Studio is ideal for monitoring industrial IoT systems, where multiple sensors continuously generate data. For example:
- System Setup: Sensors measuring temperature, vibration, and pressure connected to a local MQTT broker.
- Outcome: Real-time monitoring and anomaly detection, reducing downtime by 30%.
5.2.2 Academic Research
Educators and students use Serial Studio to visualize data in projects involving embedded systems and robotics. Example:
- Scenario: A robotics lab tracking motor RPM and battery levels.
- Result: Enhanced understanding of system behavior through clear visual feedback.
5.2.3 Environmental Monitoring
Serial Studio excels in environmental projects, such as weather stations or pollution tracking systems. It enables users to:
- Visualize temperature, humidity, and air quality data.
- Analyze trends over time via graph widgets and CSV exports.
6. Customization and Extensibility
6.1 Open-Source Development
Serial Studio is open-source, licensed under the MIT License. This allows developers to modify and extend its functionality. Contributions can include:
- Adding support for new communication protocols.
- Developing custom widgets.
- Enhancing the user interface.
Example: Adding a New Widget
Developers can extend Serial Studio by implementing a new widget in the source code. Below is a simplified example:
#include "WidgetBase.h"
class CustomWidget : public WidgetBase {
public:
CustomWidget(QWidget *parent = nullptr) : WidgetBase(parent) {
setTitle("Custom Widget");
}
void updateData(const QVariant &value) override {
// Process and display data
}
};
6.2 API and Integration
Serial Studio’s API enables seamless integration with other systems and applications. Example workflows include:
- Sending processed data to a remote server.
- Combining Serial Studio with machine learning frameworks for advanced analytics.
7. Comparison with Similar Tools
To better understand Serial Studio’s advantages, here’s a comparison with other popular data visualization tools:
| Feature | Serial Studio | Processing | MATLAB | | | | | | | Platform | Cross-platform | Cross-platform | Desktop only (mostly) | | Ease of Use | High | Moderate | Low (requires scripting) | | Customizability | Extensive | Extensive | Very Extensive | | Real-Time Data Support | Excellent | Moderate | Excellent | | Open-Source | Yes | No | No |
Serial Studio is a game-changing tool for real-time data visualization in embedded systems, IoT, and research applications. Its user-friendly design, extensive features, and open-source nature make it an essential choice for developers and educators alike.
Whether you are monitoring an IoT device, debugging a robotics project, or teaching data visualization concepts, Serial Studio provides a flexible, efficient, and intuitive solution. With continuous updates and an active community, Serial Studio is well-positioned to remain a leader in the field of data visualization.
Visit the Serial Studio GitHub Repository to download the tool and explore its capabilities today!